Variables & Data Types – Python
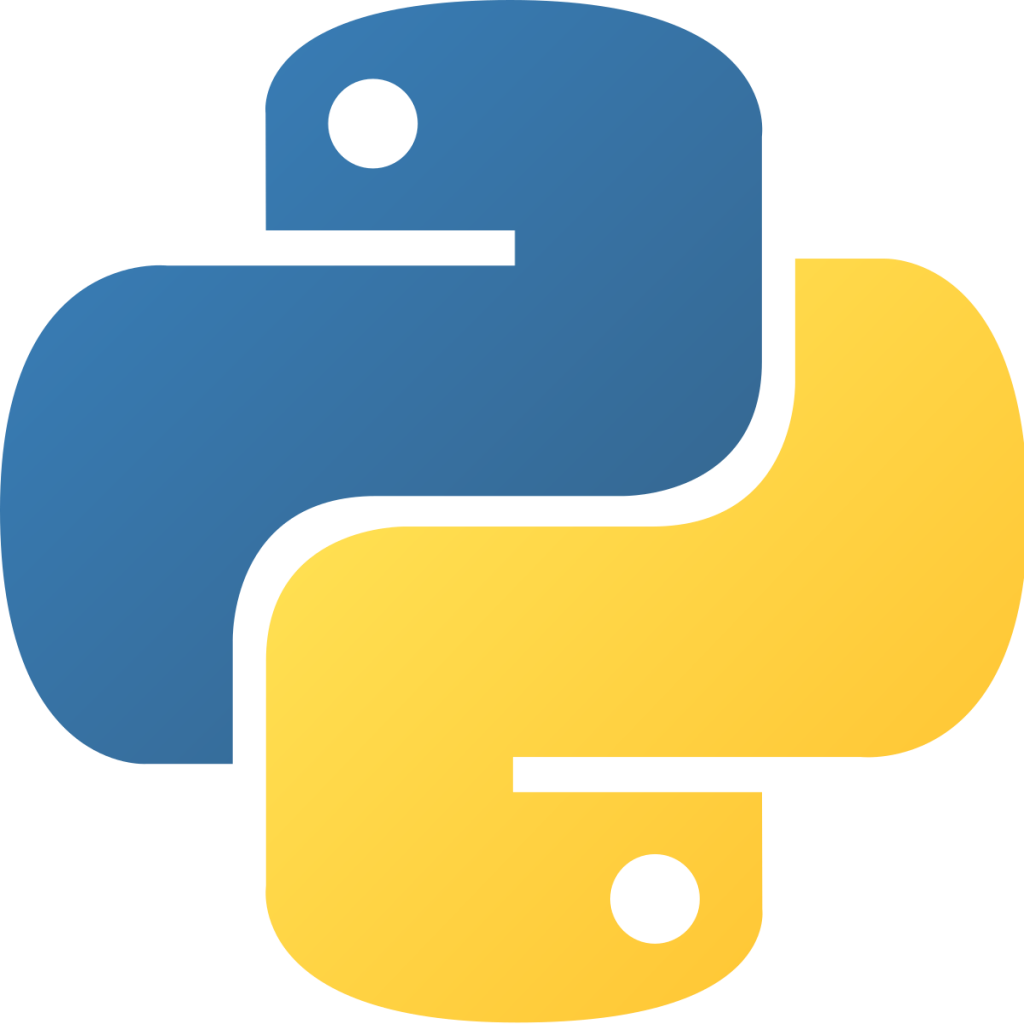
Variables
Think of a variable as a container to hold information. You can have different variables that hold different types of information. We are going to cover 4 basic data types which are: Integer, Float, String & Boolean.
Data types
Data Type | Description |
---|---|
Integer (int) | Any whole number. i.e. 3 |
Float | Any number including decimals i.e. 3.0, 12.7 |
String (str) | Any text, declared by being wrapped in quotation marks i.e. “Hello!” |
Boolean (bool) | Only has two possible values, true or false. |
Practical
To assign a data type to a variable we use the equal sign = operator. For example, see below:
# Let's say I wanted to save my name which is Paige, into a variable
# called my_name. It would look like the following
my_name = "Paige"
#Note how we use the quotation marks around Paige. This is to ensure
#that Paige is interpreted as a string.
# You may have two other questions...
# First, what's with the use of the hashtag. hashtags tell the interpretor
# that these are comments for the human, therefore don't execute this!
"""
If you'd like to write multiple lines of comments you can use three
quotation marks in a row at the start and end of your comment.
"""
# The second question you may have is, why did we name the variable
# as my_name, what's with the lower case and underscore?
# This is about style. For variables, the PEP 8 guidelines suggest
# variable names should be lowercase, with words separated by underscores
# as necessary to improve readability. Commonly, this is referred to as
# snake_case
So I have my name in the variable my_name created and it’s storing “Paige”. Now we want it to display the name. To do this we must use the print() function. A function is something that performs a specific task. We will be getting well acquainted with functions later on. For now, just know you call a function by saying its name followed by opening and closing parentheses.
Copy the code below and try it for yourself in Spyder! Feel free to replace Paige with your name.
my_name = "Paige"
print(my_name)
What if we want to print “Hello Paige!” instead? Take a look at the code below.
my_name = "Paige"
print("Hello " , my_name , "!")
We are using the comma to add the word “Hello” and an exclamation point to what is printed.
Now imagine if we someone to input their own name. We could make sure of the input() function. The input() function takes whatever is written in the console and stores that in a variable. The user has to type their name and then press enter.
my_name = input()
print("Hello " , my_name , "!")
If you run the above code, you may find it strange that the program doesn’t prompt you to type your name. Go ahead and add that now. Hint! Python executes codes sequentially from top to bottom!
Click to reveal ↓
print("Type your name here, followed by the enter button:")
my_name = input()
print("Hello " , my_name , "!")
So far we’ve been working with string data types. Let’s work with numbers. Can you use what you’ve learned so far to create two variables in which the value is inputted by the user and then add those values together?
Click to reveal ↓
You may have some code similar to the below and are frustrated that if the numbers 5 and 6 are inputted that you’re getting returned 56 and not 11!
print("Enter a number here using the number pad:")
number_one = input()
print("Enter another number here using the number pad:")
number_two = input()
print("Your numbers when added together come to:" , number_one + number_two)
Why is this? This is because the input is recording the numbers entered as strings, not integers or floats. What we need to do is declare the input as either an integer or float, depending on if we want whole numbers or decimals. We can do this using the int() or float() function which converts information inside of the parentheses into the corresponding data type. Let’s try this again with the code below
print("Enter a number here using the number pad:")
number_one = int(input())
print("Enter another number here using the number pad:")
number_two = int(input())
print("Your numbers when added together come to:", number_one + number_two)
Ta-da! When we add together 5 and 6 we should now receive the answer 11!
Section Challenge
To end this section, I’d like you to write some code that takes the input of three numbers and averages them. Hint! Python uses mathematical operators such as + for addition and / for division. Python also follows the same order of operations as in maths.
Click to reveal ↓
When programing there are many ways to achieve the same answer, don’t worry if your answer is different from mine!
print("Enter a number here using the number pad:")
number_one = int(input())
print("Enter another number here using the number pad:")
number_two = int(input())
print("Enter your final number here using the number pad:")
number_three = int(input())
print("Your numbers when averaged together come to:", (number_one + number_two + number_three)/3)
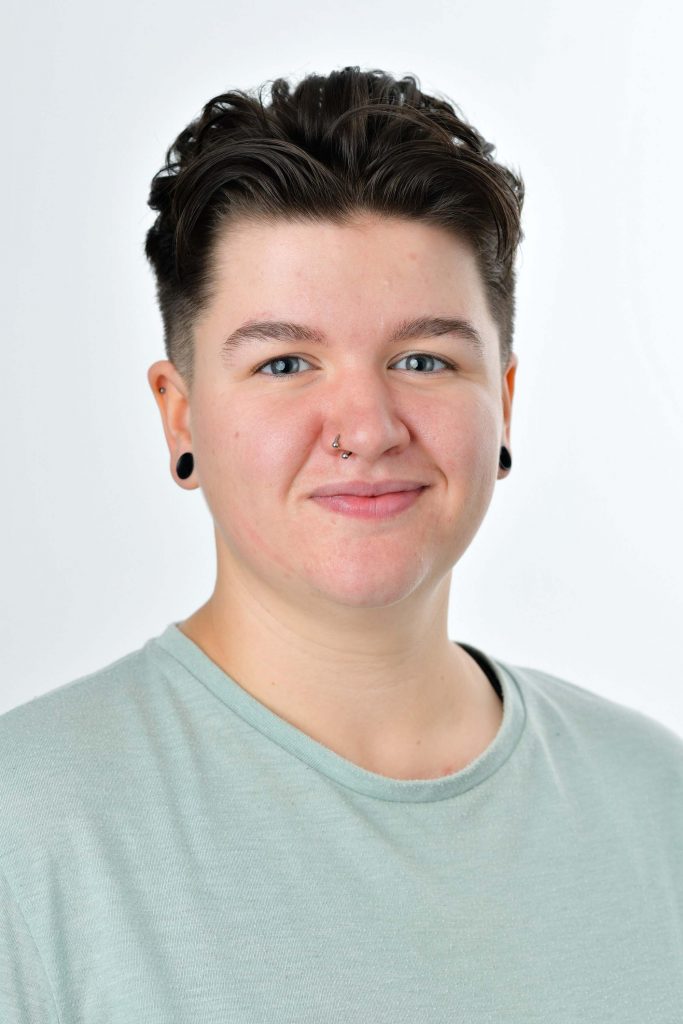
Course Author
Paige Metcalfe
c.p.metcalfe@salford.ac.uk