Data Structures – Python
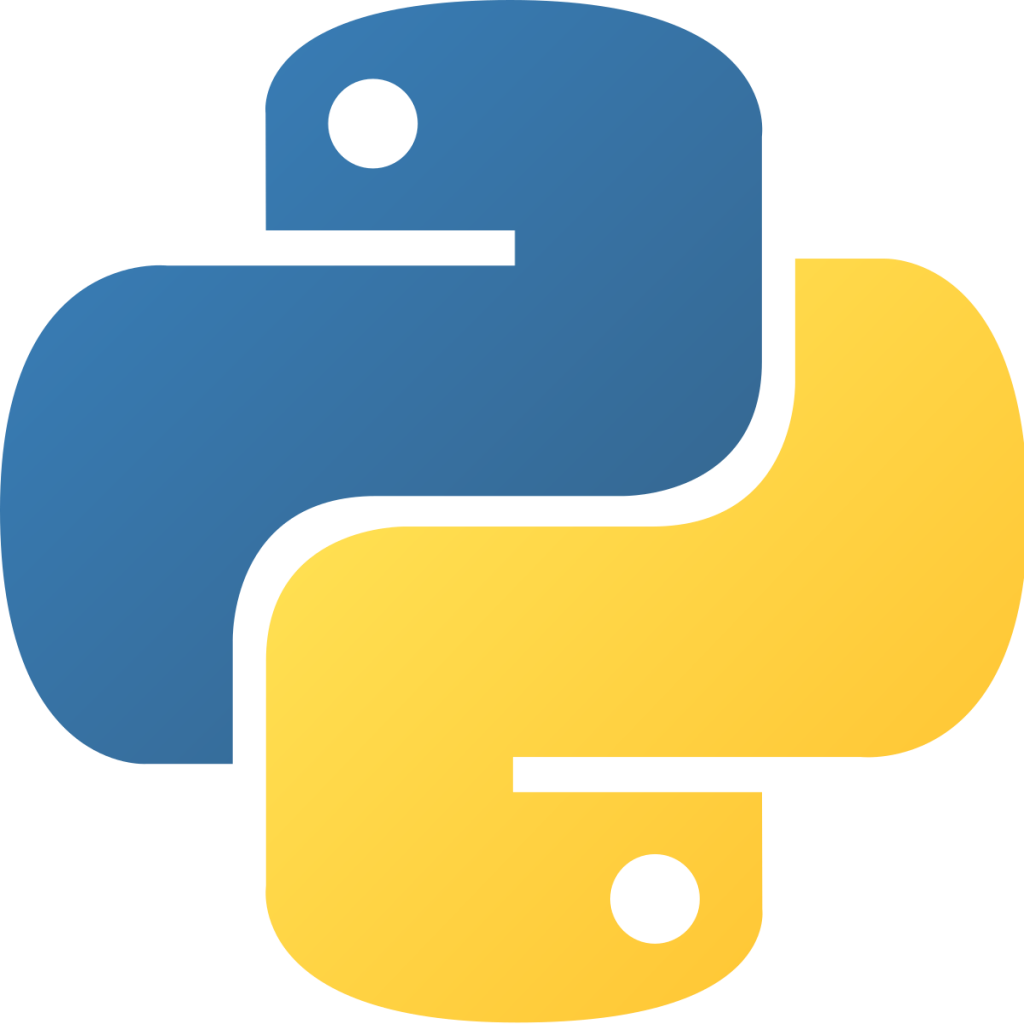
Up to this point, we have been using variables that are storing one piece of data. Usually, when working with data we want to store more than one value. Python does have the ability to work with arrays, vectors, and import excel spreadsheets or .csv files, so on and so forth!. Although we’ll get to that later in the lesson. First, we’re going to cover python’s built-in data structures which are lists, tuples, sets, and dictionaries.
this_list = ["list element 0", "list element 1", "list element 2", "list element 3"]
# note the square brackets
# additionally note how each piece of information in the list is
# refered to as an element. You may also seem them refered to as an item.
this_tuple = ("tuple element 0", "tuple element 1", "tuple element 2", "tuple element 3")
# note the paratheses
this_set = {"set element 0", "set element 1", "set element 2", "set element 3"}
# note the curly brackets
this_dictionary = {
"Key 1": "a",
"Key 2": ["b", "c", "d"], # You can even store lists, tuples and sets
"Key 3": ("e", "f", "g"), # within a dictionary
"Key 4": {"h", "i", "j"}
}
# we can use a comindation of the print() and type() function to get python
# to tell us what it is if we needed (you could also look in the variable
# explorer in spyder)
print(type(this_dictionary))
# type() will return the variable's class, it's a good tool to use!
Each data type has its own advantages and disadvantages, they can store all the basic data types we covered in a previous lesson. When deciding what data type to use, kind about the characteristics of that data type, to help you decide. The list data type gives you a lot of freedom and flexibility. However, what if this flexibility causes you to accidentally overwrite some important data? If that is a major concern, then you’d consider using a Tuple, since they are not changeable. Because they’re not changeable, tuples use less memory, meaning your program will execute faster. Lists and tuples are very common. However, sets have a more niche use case. Sets don’t have an order and don’t allow duplicates, which means they’re very suited to checking to see if the same elements are in the set or not or other similar membership tests. Dictionaries can store all the data types, it’s useful if you’d like to categorise your data but keep it within the same data frame.
Lists | Tuples | Sets | Dictionaries | |
---|---|---|---|---|
Set order? | Yes | Yes | No | Yes – in 3.7* |
Allows duplicates? | Yes | Yes | No | Depends** |
Changeable? | Yes | No | No*** | Yes |
**Dictionaries cannot have two or more keys with the same name. Elements of any key can be duplicated.
***Set items are unchangeable, but you can remove and/or add items whenever you like
These data types allow us to store multiple values in a single variable. I’ll be very honest with you, I’m biased. I’ve only ever really used Lists, and the occasionally Dictionary. So we are going to be focusing on Lists! When we start using packages, we’re going to be learning new data structures! One great thing about lists, is that we can access elements in the list via their index. For example, the first element in the list has an index of zero, because remember python is zero-indexed. Let’s combine this knowledge of being able to access data in a variable by its index using loops.
What we want to create is a program that will ask someone how many animals they can remember and then it tells them how many they remember, feel free to make your own or you can use the code below!
Make your own or use this:
remembered_animals = [] # Here we are making an empty list for the user to
# populate with their answers
print("List as many animals as you can, when you can't think of any more write 'Done':")
while True: #this creates an infinate loop since we don't know how many animals the user will remember
animal = input() #stores only the current iteration of the loop
remembered_animals.append(animal) #.append is a method that adds
# the contents of our animal variable
# to our list
if animal == "Done": #this is the exit condition of the loop
break
print("You remembered", len(remembered_animals), " animals!")
# The len() function will return how many elements
# are in the list. This is the same logic as when we
# combined the print() and the type() function previously
#now let's show what they rememberered.
#of course we COULD just write print(remembered_animals)
#But we want to print the elements of the list one by one.
#Let's use a for loop to do thing.
print("Here is what you remembered:")
for i in range(len(remembered_animals)):
# We can use the len() function to specify how long the for
# for loop. This is good practice since it allows the loop
# to be dynamic dependant on user input
print(i+1, ". ", remembered_animals[i]) # here I'm using i so it interates
# through the list.
We’re combing a lot of the things we’ve learned so far in the above code to make sure you take the time to play around and understand it.
Challenge!
What I’d like you to do, is modify the above code and make it so that when printing the elements of the array, the last element which is “Done”, is not included, since we’re using the word “Done” as a condition to exit the loop and isn’t an animal.
Possible solution: This challenge has many solutions (don’t they all), one possible solution is a method known as .pop() that removes the last element. You would use the following syntax: variable.pop()
Click to reveal ↓
Here I’m presenting two solutions, as always, don’t worry if your answer is slightly different, there are many ways to achieve the same thing!
The below code uses the .pop() method to remove the last item in the array
remembered_animals = [] # Here we are making an empty list for the user to
# populate with their answers
print("List as many animals as you can, when you can't think of any more write 'Done':")
while True: #this creates an infinate loop since we don't know how many animals the user will remember
animal = input() #stores only the current iteration of the loop
remembered_animals.append(animal) #.append is a method that adds
# the contents of our animal variable
# to our list
if animal == "Done": #this is the exit condition of the loop
break
remembered_animals.pop() #thos
print("You remembered", len(remembered_animals), " animals!")
# The len() function will return how many elements
# are in the list
print("Here is what you remembered:")
for i in range(len(remembered_animals)):
# We can use the len() function to specify how long the for
# for loop. This is good practice since it allows the loop
# to be dynamic dependant on user input
print(i+1, ". ", remembered_animals[i]) # here I'm using i so it interates
# through the list.
There is another approach that doesn’t use the .pop() method. Simply it changes the order of the loop. The loop’s order of actions is changed to only append the input when the if statement is false.
remembered_animals = [] # Here we are making an empty list for the user to
# populate with their answers
print("List as many animals as you can, when you can't think of any more write 'Done':")
while True: #this creates an infinate loop since we don't know how many animals the user will remember
animal = input() #stores only the current iteration of the loop
if animal == "Done": #this is the exit condition of the loop
break
remembered_animals.append(animal) # here we're changed the loop order
# so the input is only appended if
# the condition of the if statement
# is false
print("You remembered", len(remembered_animals), " animals!")
# The len() function will return how many elements
# are in the list
#now let's show what they rememberered.
#of course we COULD just write print(remembered_animals)
#But we want to print the elements of the list one by one.
#Let's use a for loop to do thing.
print("Here is what you remembered:")
for i in range(len(remembered_animals)):
# We can use the len() function to specify how long the for
# for loop. This is good practice since it allows the loop
# to be dynamic dependant on user input
print(i+1, ". ", remembered_animals[i]) # here I'm using i so it interates
# through the list.
This exercise is a really great way to show the many ways you can achieve the same output! Next, I’d like you to remove the 2nd user answer given to your list, and print the list. Then ask the user if they can remember what their 2nd answer was. Give it a try!
Hint: When using the .pop() method you can indicate the index you want to remove inside of the paratheses. Additionally, you can combine the .pop() method with a variable assignment and save the ‘popped’ item into a variable.
Click to reveal ↓
Don’t worry if your answer is slightly different, there are many ways to achieve the same thing!
remembered_animals = [] # Here we are making an empty list for the user to
# populate with their answers
print("List as many animals as you can, when you can't think of any more write 'Done':")
while True: #this creates an infinate loop since we don't know how many animals the user will remember
animal = input() #stores only the current iteration of the loop
remembered_animals.append(animal) #.append is a method that adds
# the contents of our animal variable
# to our list
if animal == "Done": #this is the exit condition of the loop
break
remembered_animals.pop() #thos
print("You remembered", len(remembered_animals), " animals!")
# The len() function will return how many elements
# are in the list
if len(remembered_animals) >= 2: # here we are checking to see if the user gave 2 or more answers
print("Let's remove the second animal you gave in your list \n \n ") #here I've used \n to create a new line to make things look tidier!
second_answer = remembered_animals.pop(1) #remember python is zero indexed! # additionally, we're saving the item to it's own variable
print("Here is what you remembered, execpt for your second answer:")
for i in range(len(remembered_animals)):
# We can use the len() function to specify how long the for
# for loop. This is good practice since it allows the loop
# to be dynamic dependant on user input
print("> ", remembered_animals[i]) # here I'm using i so it interates
# through the list.
print("Can you remember what your second answer was?")
question_answer = input() #Taking input for the question
if question_answer == second_answer: #checking to see if the user input is the same as the popped elemebt
print("That's correct!") #if it is, tell them it's correct
else: #if it's not, tell them it's incorrect
print("That's incorrect.")
else:
pass
#look at the nesting in the above if/else statement and how it nests a loop and another if/else statement!
Certainly, at this point, and before now, you should be proud of how far you’ve come!
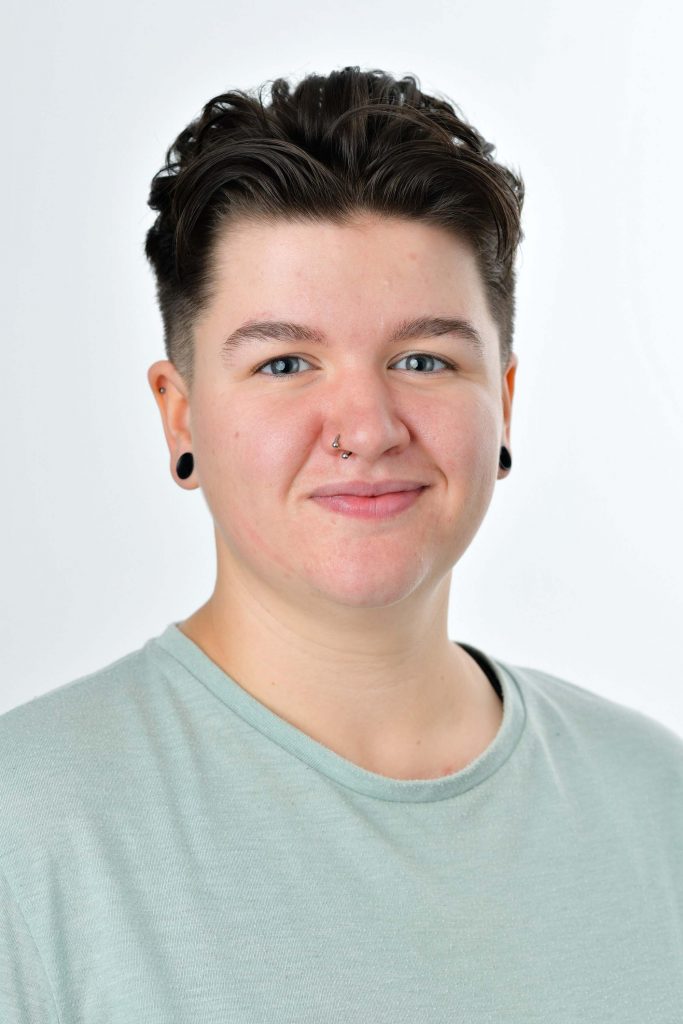
Course Author
Paige Metcalfe
c.p.metcalfe@salford.ac.uk