Operators & Conditionals – Python
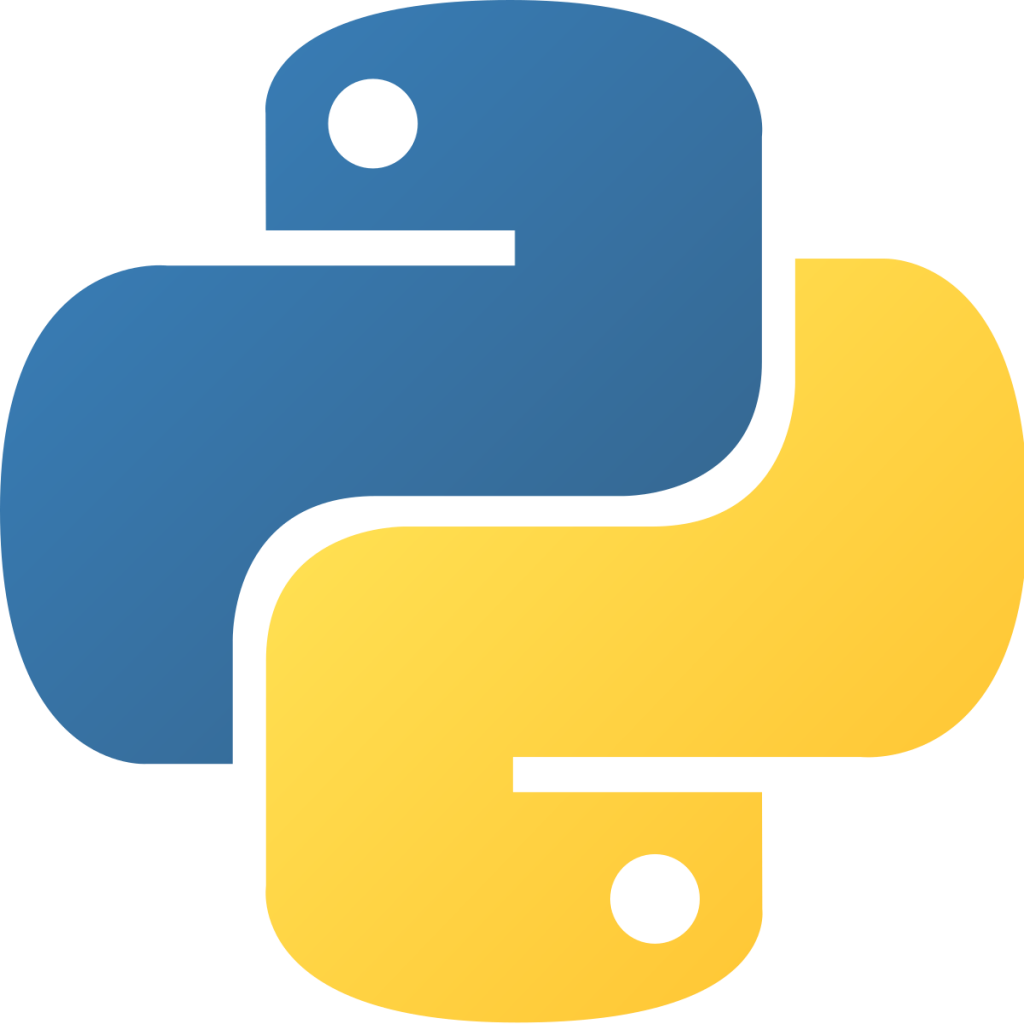
Operators
In the last lesson, you were introduced to the idea that you can do arithmetic in python. Let’s explore that some more. Look at the code below to see some arithmetic operators, feel free to play around with the code below
x = 10 #Feel free to change this
y = 5 #Again, feel free to change
print(x+y) #Addition
print(x-y) #Substraction
print(x*y) #Multiplication
print(x/y) #Division
print(x%y) #Modulus
print(x**y) #Exponentiation
print(x//y) #Floor division
Much like when we assign a value using the equal = operator, we have other ways of assigning values. For example.
x = 10 #We assign x the value of ten
x += 5 #Here we now assigning x the value of x + 5.
print(x) #This will print the NEW value of x which is 15.
The same logic applies to the other arithmetic operators. Ensure you’re putting the arithmetic operator first followed by the equal = assignment operator with no spaces. Give it a try with the rest.
More operators by now we’re using them with conditionals.
So it’s cool we can use arithmetic operators, and it’s also cool we can combine the arithmetic operators with the assignment operator.
Now there are going to be times when we only want the code to do something if a condition is met. Let’s say we only want to run the code if the variable x is bigger than the variable y. Here is how that would look.
x = 10
y = 5
if x > y: #note the if statement and colon
print("x is greater than y") #note the indentation
print("The code is done now.")
The above code points out the if statement, colon, and indentation. In python the word if is a keyword. Keywords have a reserved meaning in the python language, so do not use them as variable names. The if statement pretty much does as you’d expect, if something is true or false do something. Wait, it’s the true or false idea something we’ve heard before? Yes, it is! It’s known as boolean logic. What about the colon and indentation, well that is ensuring you have the correct syntax for your code to run. Let’s say we make the variable y bigger than variable x, what do you think would happen? If you guessed that the indented part of the code doesn’t run then you’d be right. Give this concept a try with the other comparison operators.
Comparison operator | Description |
---|---|
== | The same as |
!= | Not the same as |
> | Greater than |
< | Less than |
>= | Greater than or equal to |
<= | Less than or equal to |
All of the comparison operators can handle floats and integers. However, not all of the comparison operators can work with strings. You can only use the same as == and not the same as != comparison operators with strings. Although, that’s all we need if we can to make a simple quiz.
print("Quiz time: What is the surname of the researcher who is known for The Strange Situation? Write your answer...")
answer = input()
if answer == "Ainsworth":
print("This is correct!")
print("The quiz is over.")
The quiz asks, what is the surname of the researcher who is known for The Strange Situation? The correct answer the quiz program is looking for is Ainsworth. However, if the user inputs the wrong answer, then it doesn’t tell them. Let’s fix that using an else statement.
print("Quiz time: What is the surname of the researcher who is known for The Strange Situation? Write your answer...")
answer = input()
if answer == "Ainsworth":
print("This is correct!")
else: #note how this is aligned with the if statement that came prior
print("This is incorrect.") #again for else statments we intend
#what we want to happen
print("The quiz is over.")
The else statement is a catch-all, which runs if any previous conditions are not met. Now, we don’t always want to sort into two groups. What if someone confuses Ainsworth’s work with another popular psychologist at the time, such as Bowlby. We can let them know that they are close. Well, we can do this using an elif statement. The elif statement goes between the if and the else statement and says if the previous conditions aren’t met, try this one. Let’s try it.
print("Quiz time: What is the surname of the researcher who known for The Strange Situation? Write your answer...")
answer = input()
if answer == "Ainsworth":
print("This is correct!")
elif answer == "Bowlby": #Don't forget the colon!
print("Incorrect. Bowlby, is an attachment theorist but he did not develop the strange situation! ") #Don't forget the indentation
else:
print("This is incorrect.")
print("The quiz is over.")
Take some time to play around with this idea! Try creating something that uses the not the same as operator !=.
Multiple Conditions!
Let’s say we only want to execute some code if two conditions are met. We’ll go with the scenario where we’re screening participants for a research project. In order to be eligible to participate, they have to be 18 or over and they have to be right-handed.
print("We'd like to ask you some questions to see if you're a suitable participant.")
print("Please enter using the keypad your age")
age = int(input())
print("Are you right handed? Please indicate by typing Yes or No")
hand = input()
if age >= 18 and hand == "Yes":
print("Great, you're eligible to take part!")
else:
print("unfortunately, you're not eligible to take part")
print("End of questions.")
You can see above that you can use the and operator to specify that two conditions must be met in order for the following indented code to run. Python also has an or operator that can be used in the same way the and operator is used. The or operator runs the following indented code if one or the other conditions is met. Using an elif statement and the or operator, I’d like you to add a condition that will tell the user they only meet one of the conditions.
Click to reveal ↓
Don’t worry if your answer is slightly different, there are many ways to achieve the same thing!
print("We'd like to ask you some questions to see if you're a suitable participant.")
print("Please enter using the keypad your age")
age = int(input())
print("Are you right handed? Please indicate by typing Yes or No")
hand = input()
if age >= 18 and hand == "Yes":
print("Great, you're eligible to take part!")
elif age > 18 or hand == "Yes":
print("Unfortunately you don't meet both of the requirements.")
else:
print("unfortunately, you're not eligible to take part")
print("End of questions.")
Challenge!
For the program above, I’d like you to write some code. What I’d like the code to do is the following. If someone enters an age below 18 and they’re right-handed, I want the program to tell them how many years they have to wait to be old enough to participate.
Click to reveal ↓
Don’t worry if your answer is slightly different, there are many ways to achieve the same thing!
print("We'd like to ask you some questions to see if you're a suitable participant.")
print("Please enter using the keypad your age")
age = int(input())
print("Are you right handed? Please indicate by typing Yes or No")
hand = input()
if age >= 18 and hand == "Yes":
print("Great, you're eligible to take part!")
elif age > 18 or hand == "Yes":
print("Unfortunately you don't meet both of the requirments.")
else:
print("Unfortunately, you're not eligible to take part")
if age < 18 and hand == "Yes": #I've put this is an entirely different
#chain of if and else statments
remaining = 18 - age #This is where we calculate how many years
print("You have ", remaining, "years until you're old enough to participate.")
print("End of questions.")
Add on:
What do you do if none of your conditions are met and you want the program to do nothing? If this occurs then you’d use a pass statement. The pass statement basically say, “do nothing!”, it’s a great placeholder since empty code isn’t allowed in if, elif, and else statements.
In the next session, we’ll be covering loops!
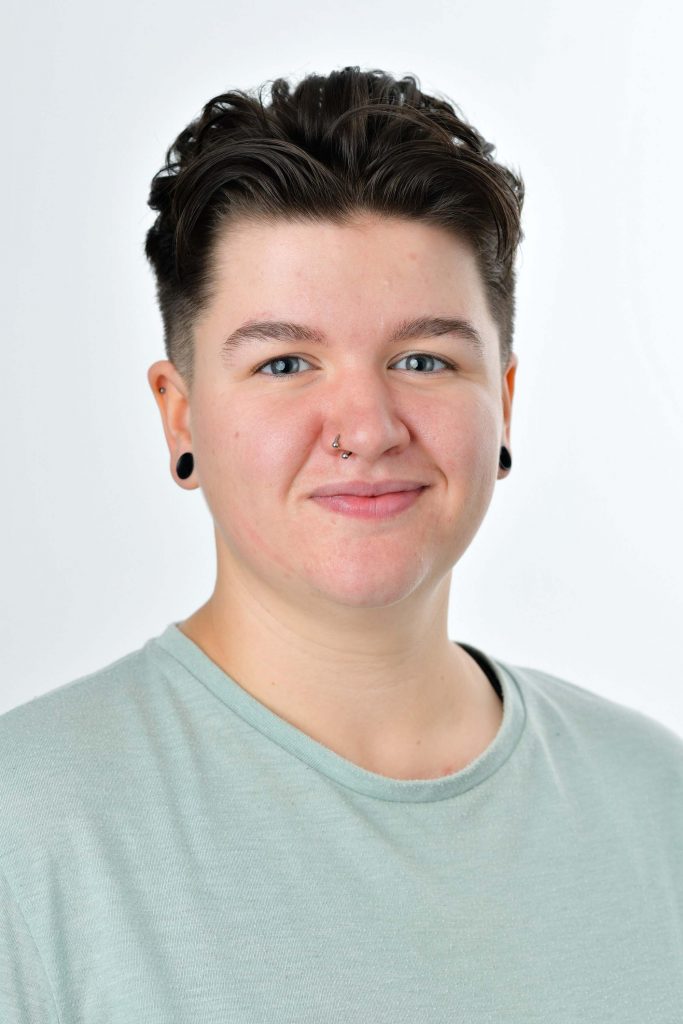
Course Author
Paige Metcalfe
c.p.metcalfe@salford.ac.uk