Loops – Python
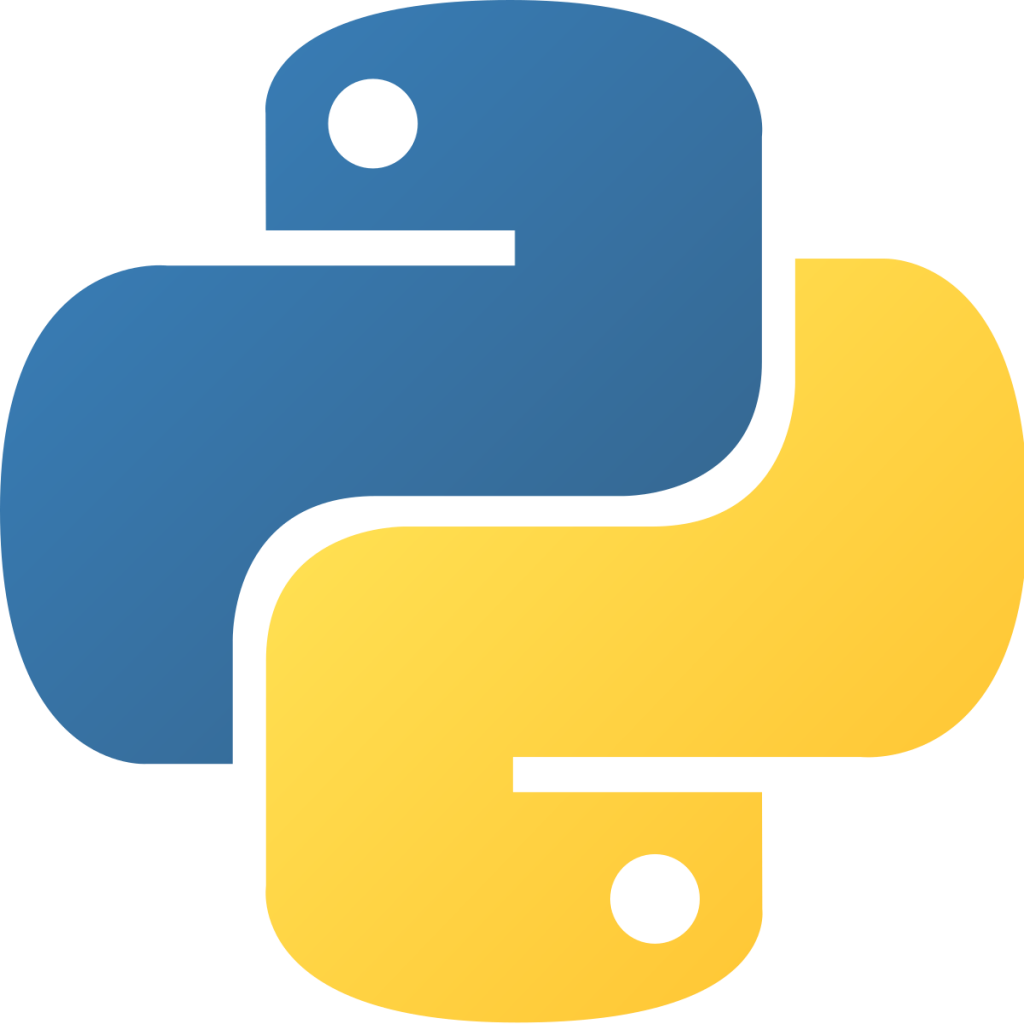
Loops allow up to repeat code and iterate over large data sets. The latter, we’ll be getting more into in the next session. Loops come in two varieties, you have while loops and for loops. While loops will run the code while a condition is met. For loops will run the code for a specified number of times.
While Loops
We’re going to create some code that allows the user to guess a number between 1 – 10, however, the user only has 3 guesses. Let’s run the code below to start off.
i=1 #usually in loops the letter i is used, i stands for iterate (or index!)
while i <= 3: # What we're saying here is run this code while i is less than or
# equal to three - this is our three guesses
# note the syntax! while is a keyword is python!
print("Guess a number between 1 and 10 using the number pad:")
guess = int(input())
What happened when you ran the code? You’ll have found it would continuously loop indefinitely. This is because, in our loop, we’re not increasing the value of i by 1. Go ahead and see if you can solve this problem.
Click to reveal ↓
Don’t worry if your answer is slightly different, there are many ways to achieve the same thing!
i=1
while i <= 3:
print("Guess a number between 1 and 10 using the number pad:")
guess = int(input())
i += 1 #Adding this line means we now get three guesses, woo!
Great, we have three guesses in our program now. Next, we need to get the program to generate a number between 1 and 10 then tell us if we’re right and what the right number is if we don’t guess it. We’ll start off by randomly generating a number.
import random #Base python doesn't have everything we need, at times we need
#to import packages, that have useful things for us to use.
# We'll be going over packages in future lessons.
# For now just know they exisit.
number = random.randint(1,10) #the random.randint() is from the random that we
# imported above. All this is doing is
#generating a number between 1 and 10
i=1
print("The correct answer is ", number)
while i <= 3:
print("Guess a number between 1 and 10 using the number pad:")
guess = int(input())
i += 1
Now the program is generating a number. For testing purposes, I’ve added the program to print the correct answer before we guess so we can test it. Next, we need the program to tell the user if their guess is right or wrong. For you to do! I’d like you to add an if statement to the code above to tell the user if they’ve guessed correctly.
Click to reveal ↓
Don’t worry if your answer is slightly different, there are many ways to achieve the same thing!
import random
number = random.randint(1,10)
i=1
print("The correct answer is ", number)
while i <= 3:
print("Guess a number between 1 and 10 using the number pad:")
guess = int(input())
if guess == number:
print("You are right!")
i += 1
You may notice the program still gives us all our guesses even if we guess the number correctly the first time (which for now we can since the program tells us the correct answer). We’re going to get the program to exit out of the loop early when the correct answer is given. To do this we’re going to use the break statement. Our program now looks like this.
import random
number = random.randint(1,10)
i=1
print("this is the answer ", number)
while i <= 3:
print("Guess a number between 1 and 10 using the number pad:")
guess = int(input())
if guess == number: # Note that this if statement is nested in the while loop
print("You are right!")
break
i += 1
Give it a try. The program should stop if you guess the right number. In addition, to break statements that stop the program if the conditions are met, we also can continue statements that continue if the conditions are met. We’re not using continue statements in this example but they are useful tools to remember!
Now I’d like you to add an elif statement so the program will tell the user when an incorrect number is guessed and how many guesses they have remaining.
Click to reveal ↓
Don’t worry if your answer is slightly different, there are many ways to achieve the same thing!
import random
number = random.randint(1,10)
i=1
print("this is the answer ", number)
while i <= 3:
print("Guess a number between 1 and 10 using the number pad:")
guess = int(input())
if guess == number:
print("You are right!")
break
elif guess != number:
remaining = 3 - i
print("Incorrect! You have ", remaining, " attempts remaining.")
i += 1
The last part of our program is to tell the user what they number is if they guessed it wrong with their 3 guesses! To do this, we use an else statement that is in line with the while keyword. Give it a go!
Click to reveal ↓
Don’t worry if your answer is slightly different, there are many ways to achieve the same thing!
import random
number = random.randint(1,10)
i=1
# print("this is the answer ", number) # this line of code is commented out
# we only needed to print the answer
# when we were testing
while i <= 3:
print("Guess a number between 1 and 10 using the number pad:")
guess = int(input())
if guess == number:
print("You are right!")
break
elif guess != number:
remaining = 3 - i
print("Incorrect! You have ", remaining, " attempts remaining.")
i += 1
else:
print("You've run out of attempts, the correct number was", number)
For Loops
Let’s move onto for loops that run for a number of iterations. The syntax is the same as while loops, we just replace while with for. In our for loop, we’re going to be using the range() function, which allows us to specify the number of times the code will run.
for i in range(10):
print(i)
Note how we don’t have to specify a value for i when we’re using for loops. Although when you run the code, notice how the program prints the numbers 0 through to 9. This is because python uses zero-indexing. The very first time the for loop runs, python will assume i = 0 unless you specify otherwise. Using the range() function we can specify we want the numbers 1 to 10 by writing range(1,11). The program needs range(1,11) by standing at 1 and stopping at 11, therefore 11 is not included. Give it a try using the code below.
for i in range(1, 11):
print(i)
By default, python will increment the value i but 1. You can change this by adding a third argument to the range() function. We will be covering arguments, for now just know it’s the values we place in the parentheses of the function. To change the increments from the default of 1 to your specified number, I’m going to use 2, try the following code.
for i in range(1, 11, 2):
print(i)
Session Challenge!
I’d like you to create a program that simulates a coin toss. Your program will ask the user how many times they would like to toss a coin. Then simulate a coin toss as many times as the user requested. To finish with, the program will tell the users how tosses they were in addition to how many heads and how many tails. You can use the code below to get started.
import random
print("How many times would you like to toss a coin?")
for i in range(#add here):
flip = random.randint(0, 1) #We're getting the code to generate randomly either a 0 or a 1. One value will represent heads the other value will represent tails.
#hint: you'll need to use if statements
Click to reveal ↓
This time I’ve provided two answers depending on how you’ve approached the range() function. As always, don’t worry if your answer is slightly different, there are many ways to achieve the same thing!
import random
print("How many times would you like to toss a coin?")
tosses = int(input()) #Taking the amount of times you user would like to toss the coin
heads = 0 #we're going to use these varibles to act as a counter for how many
tails = 0 # heads and how many tails.
for i in range(tosses):
flip = random.randint(0, 1) # We're getting the code to generate
# randomly either a 0 or a 1.
if flip == 0: # in my code if the code randomly generates a 0, then we're considering that a heads
print("Heads!")# if this condition is met then we're prints heads to show what side of the coin
heads += 1 # and we're adding one to our heads variable
else: #if you wanted you could of wrote elif flip == 1: however, since this is a binary else works as well
print("Tails!") #tells the user a tails was flipped
tails += 1 #adds 1 to the tails counter
print("The was a total of ", tosses, " tosses.", heads, "heads and ", tails, "tails.")
Alternate answer if you chose to specify the range.
import random
print("How many times would you like to toss a coin?")
tosses = int(input())+1 #We add one since we want to include
#the value the user specifies
heads = 0 #we're going to use these varibles to act as a counter
tails = 0 # for how many heads and how many tails.
for i in range(1, tosses):
flip = random.randint(0, 1) #We're getting the code to generate
# randomly either a 0 or a 1.
if flip == 0: # in my code if the code randomly generates a 0,
# then we're considering that a heads
print("Heads!") # if this condition is met then we're prints
# heads to show what side of the coin
heads += 1 # and we're adding one to our heads variable
else: #if you wanted you could of wrote elif flip == 1: however,
# since this is a binary else works as well
print("Tails!") # tells the user a tails was flipped
tails += 1 # adds 1 to the tails counter
print("The was a total of ", tosses - 1, " tosses.", heads, "heads and ", tails, "tails.") #we have to minus off the one we added previously
#to ensure we display the correct number
An important point to remember!
In all the examples of loops on this page, we’ve used i. You can replace i with and letter you would like, or a variable.
In the next lesson, we’ll be taking a look at how to store and interact with larger data sets.
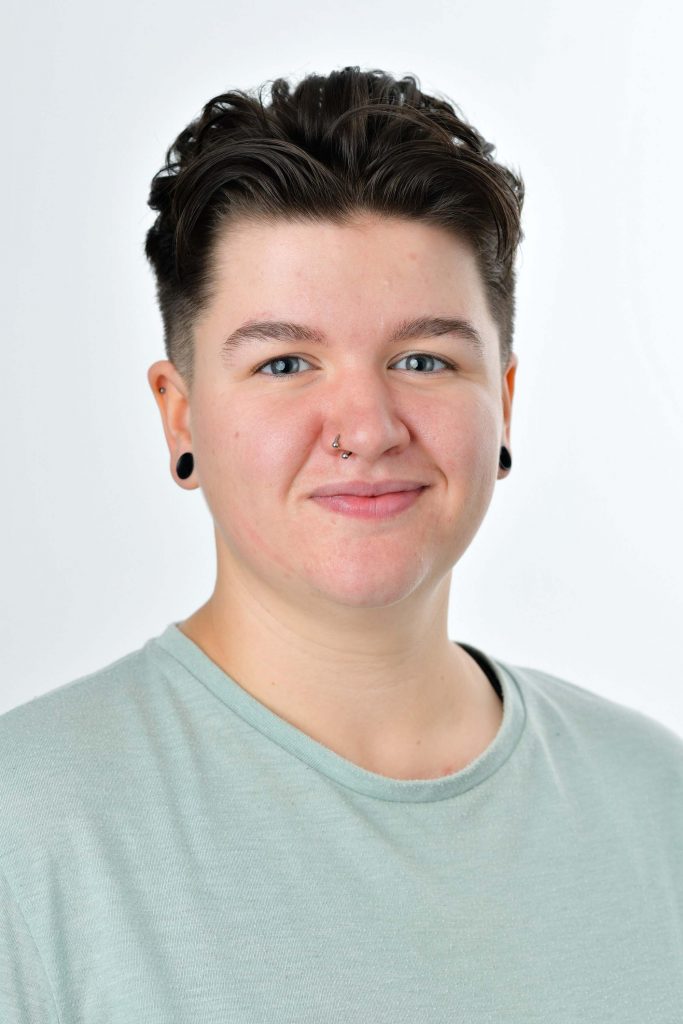
Course Author
Paige Metcalfe
c.p.metcalfe@salford.ac.uk